Commit 1b04ffe
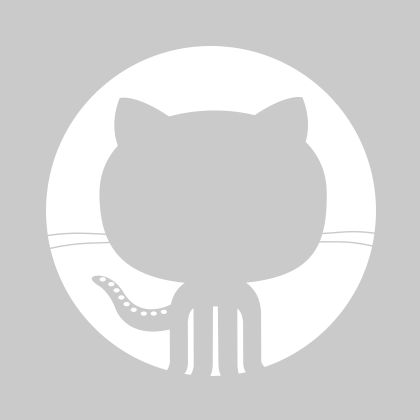
Jonathan Dowland
1 parent b2619cf commit 1b04ffe
File tree
5 files changed
+453
-274
lines changed- jdk
- src/linux/classes/jdk/internal/platform
- cgroupv1
- cgroupv2
- test/jdk/internal/platform/cgroup
5 files changed
+453
-274
lines changedOriginal file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
26 | 26 |
| |
27 | 27 |
| |
28 | 28 |
| |
29 |
| - | |
| 29 | + | |
| 30 | + | |
30 | 31 |
| |
31 | 32 |
| |
32 | 33 |
| |
33 | 34 |
| |
34 | 35 |
| |
35 |
| - | |
| 36 | + | |
36 | 37 |
| |
37 | 38 |
| |
38 | 39 |
| |
39 | 40 |
| |
| 41 | + | |
| 42 | + | |
| 43 | + | |
40 | 44 |
| |
41 | 45 |
| |
42 | 46 |
| |
43 | 47 |
| |
44 | 48 |
| |
45 | 49 |
| |
46 | 50 |
| |
47 |
| - | |
| 51 | + | |
48 | 52 |
| |
49 | 53 |
| |
50 | 54 |
| |
51 |
| - | |
| 55 | + | |
52 | 56 |
| |
53 | 57 |
| |
54 | 58 |
| |
55 |
| - | |
| 59 | + | |
56 | 60 |
| |
57 | 61 |
| |
58 | 62 |
| |
| 63 | + | |
| 64 | + | |
| 65 | + | |
| 66 | + | |
| 67 | + | |
| 68 | + | |
| 69 | + | |
| 70 | + | |
| 71 | + | |
| 72 | + | |
| 73 | + | |
| 74 | + | |
| 75 | + | |
| 76 | + | |
| 77 | + | |
| 78 | + | |
| 79 | + | |
| 80 | + | |
| 81 | + | |
| 82 | + | |
| 83 | + | |
| 84 | + | |
| 85 | + | |
| 86 | + | |
| 87 | + | |
| 88 | + | |
| 89 | + | |
| 90 | + | |
| 91 | + | |
| 92 | + | |
| 93 | + | |
| 94 | + | |
| 95 | + | |
| 96 | + | |
| 97 | + | |
| 98 | + | |
| 99 | + | |
| 100 | + | |
| 101 | + | |
| 102 | + | |
| 103 | + | |
| 104 | + | |
| 105 | + | |
| 106 | + | |
| 107 | + | |
| 108 | + | |
59 | 109 |
| |
60 | 110 |
| |
61 | 111 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
26 | 26 |
| |
27 | 27 |
| |
28 | 28 |
| |
| 29 | + | |
| 30 | + | |
29 | 31 |
| |
| 32 | + | |
30 | 33 |
| |
31 | 34 |
| |
32 | 35 |
| |
33 | 36 |
| |
| 37 | + | |
34 | 38 |
| |
35 | 39 |
| |
36 | 40 |
| |
| |||
66 | 70 |
| |
67 | 71 |
| |
68 | 72 |
| |
69 |
| - | |
| 73 | + | |
70 | 74 |
| |
71 |
| - | |
| 75 | + | |
72 | 76 |
| |
73 | 77 |
| |
74 | 78 |
| |
75 | 79 |
| |
76 | 80 |
| |
77 |
| - | |
| 81 | + | |
78 | 82 |
| |
79 | 83 |
| |
| 84 | + | |
| 85 | + | |
80 | 86 |
| |
81 | 87 |
| |
82 | 88 |
| |
| |||
95 | 101 |
| |
96 | 102 |
| |
97 | 103 |
| |
| 104 | + | |
98 | 105 |
| |
99 |
| - | |
| 106 | + | |
| 107 | + | |
| 108 | + | |
100 | 109 |
| |
101 | 110 |
| |
102 |
| - | |
| 111 | + | |
103 | 112 |
| |
104 | 113 |
| |
105 | 114 |
| |
106 | 115 |
| |
107 |
| - | |
108 |
| - | |
| 116 | + | |
| 117 | + | |
| 118 | + | |
| 119 | + | |
| 120 | + | |
| 121 | + | |
| 122 | + | |
| 123 | + | |
| 124 | + | |
| 125 | + | |
| 126 | + | |
| 127 | + | |
| 128 | + | |
| 129 | + | |
| 130 | + | |
| 131 | + | |
| 132 | + | |
| 133 | + | |
| 134 | + | |
109 | 135 |
| |
110 | 136 |
| |
111 | 137 |
| |
| |||
136 | 162 |
| |
137 | 163 |
| |
138 | 164 |
| |
139 |
| - | |
140 |
| - | |
| 165 | + | |
| 166 | + | |
141 | 167 |
| |
142 | 168 |
| |
143 | 169 |
| |
144 |
| - | |
145 |
| - | |
146 |
| - | |
147 |
| - | |
| 170 | + | |
| 171 | + | |
| 172 | + | |
| 173 | + | |
| 174 | + | |
| 175 | + | |
| 176 | + | |
| 177 | + | |
| 178 | + | |
| 179 | + | |
| 180 | + | |
| 181 | + | |
| 182 | + | |
| 183 | + | |
| 184 | + | |
| 185 | + | |
| 186 | + | |
| 187 | + | |
| 188 | + | |
| 189 | + | |
| 190 | + | |
148 | 191 |
| |
| 192 | + | |
| 193 | + | |
| 194 | + | |
| 195 | + | |
149 | 196 |
| |
150 |
| - | |
| 197 | + | |
| 198 | + | |
| 199 | + | |
| 200 | + | |
| 201 | + | |
| 202 | + | |
151 | 203 |
| |
152 | 204 |
| |
153 | 205 |
| |
154 |
| - | |
155 |
| - | |
156 |
| - | |
157 |
| - | |
158 |
| - | |
159 |
| - | |
160 |
| - | |
161 |
| - | |
162 |
| - | |
163 |
| - | |
164 |
| - | |
165 |
| - | |
166 |
| - | |
167 |
| - | |
168 |
| - | |
169 |
| - | |
170 |
| - | |
171 |
| - | |
172 |
| - | |
173 |
| - | |
174 |
| - | |
175 |
| - | |
176 |
| - | |
| 206 | + | |
| 207 | + | |
| 208 | + | |
| 209 | + | |
| 210 | + | |
| 211 | + | |
| 212 | + | |
| 213 | + | |
| 214 | + | |
| 215 | + | |
| 216 | + | |
| 217 | + | |
| 218 | + | |
| 219 | + | |
| 220 | + | |
| 221 | + | |
| 222 | + | |
| 223 | + | |
| 224 | + | |
| 225 | + | |
| 226 | + | |
| 227 | + | |
| 228 | + | |
| 229 | + | |
| 230 | + | |
| 231 | + | |
| 232 | + | |
| 233 | + | |
| 234 | + | |
| 235 | + | |
| 236 | + | |
| 237 | + | |
| 238 | + | |
| 239 | + | |
| 240 | + | |
| 241 | + | |
| 242 | + | |
| 243 | + | |
| 244 | + | |
| 245 | + | |
| 246 | + | |
| 247 | + | |
| 248 | + | |
| 249 | + | |
| 250 | + | |
| 251 | + | |
| 252 | + | |
| 253 | + | |
| 254 | + | |
| 255 | + | |
| 256 | + | |
| 257 | + | |
| 258 | + | |
| 259 | + | |
| 260 | + | |
| 261 | + | |
| 262 | + | |
| 263 | + | |
| 264 | + | |
| 265 | + | |
| 266 | + | |
| 267 | + | |
| 268 | + | |
| 269 | + | |
| 270 | + | |
| 271 | + | |
| 272 | + | |
| 273 | + | |
| 274 | + | |
| 275 | + | |
| 276 | + | |
| 277 | + | |
| 278 | + | |
| 279 | + | |
| 280 | + | |
| 281 | + | |
| 282 | + | |
| 283 | + | |
| 284 | + | |
| 285 | + | |
| 286 | + | |
| 287 | + | |
| 288 | + | |
| 289 | + | |
| 290 | + | |
| 291 | + | |
| 292 | + | |
| 293 | + | |
| 294 | + | |
| 295 | + | |
| 296 | + | |
| 297 | + | |
| 298 | + | |
| 299 | + | |
| 300 | + | |
| 301 | + | |
| 302 | + | |
| 303 | + | |
| 304 | + | |
| 305 | + | |
| 306 | + | |
| 307 | + | |
| 308 | + | |
| 309 | + | |
| 310 | + | |
| 311 | + | |
| 312 | + | |
| 313 | + | |
| 314 | + | |
| 315 | + | |
| 316 | + | |
| 317 | + | |
| 318 | + | |
| 319 | + | |
| 320 | + | |
| 321 | + | |
| 322 | + | |
| 323 | + | |
| 324 | + | |
| 325 | + | |
| 326 | + | |
| 327 | + | |
| 328 | + | |
| 329 | + | |
| 330 | + | |
| 331 | + | |
| 332 | + | |
| 333 | + | |
| 334 | + | |
| 335 | + | |
| 336 | + | |
| 337 | + | |
| 338 | + | |
| 339 | + | |
| 340 | + | |
| 341 | + | |
| 342 | + | |
| 343 | + | |
| 344 | + | |
| 345 | + | |
| 346 | + | |
177 | 347 |
| |
178 | 348 |
| |
179 | 349 |
| |
180 | 350 |
| |
181 | 351 |
| |
182 | 352 |
| |
183 | 353 |
| |
| 354 | + | |
184 | 355 |
| |
185 | 356 |
| |
186 | 357 |
| |
187 | 358 |
| |
188 |
| - | |
| 359 | + | |
| 360 | + | |
189 | 361 |
| |
190 | 362 |
| |
191 | 363 |
| |
192 | 364 |
| |
| 365 | + | |
193 | 366 |
| |
194 | 367 |
| |
195 | 368 |
| |
| |||
207 | 380 |
| |
208 | 381 |
| |
209 | 382 |
| |
| 383 | + | |
| 384 | + | |
| 385 | + | |
| 386 | + | |
210 | 387 |
| |
211 | 388 |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
25 | 25 |
| |
26 | 26 |
| |
27 | 27 |
| |
28 |
| - | |
29 |
| - | |
30 |
| - | |
31 |
| - | |
32 |
| - | |
| 28 | + | |
33 | 29 |
| |
| 30 | + | |
34 | 31 |
| |
35 | 32 |
| |
36 |
| - | |
37 | 33 |
| |
38 | 34 |
| |
39 | 35 |
| |
| |||
42 | 38 |
| |
43 | 39 |
| |
44 | 40 |
| |
45 |
| - | |
46 | 41 |
| |
47 |
| - | |
| 42 | + | |
48 | 43 |
| |
49 | 44 |
| |
50 | 45 |
| |
51 |
| - | |
52 |
| - | |
53 |
| - | |
| 46 | + | |
54 | 47 |
| |
55 |
| - | |
| 48 | + | |
| 49 | + | |
| 50 | + | |
| 51 | + | |
| 52 | + | |
| 53 | + | |
| 54 | + | |
| 55 | + | |
| 56 | + | |
| 57 | + | |
| 58 | + | |
| 59 | + | |
| 60 | + | |
| 61 | + | |
| 62 | + | |
| 63 | + | |
| 64 | + | |
| 65 | + | |
| 66 | + | |
56 | 67 |
| |
57 | 68 |
| |
58 | 69 |
| |
59 |
| - | |
| 70 | + | |
60 | 71 |
| |
61 | 72 |
| |
62 |
| - | |
63 |
| - | |
64 |
| - | |
65 |
| - | |
66 |
| - | |
67 |
| - | |
68 |
| - | |
69 |
| - | |
70 |
| - | |
| 73 | + | |
| 74 | + | |
| 75 | + | |
| 76 | + | |
71 | 77 |
| |
72 |
| - | |
73 |
| - | |
74 |
| - | |
75 |
| - | |
76 |
| - | |
77 |
| - | |
78 |
| - | |
79 |
| - | |
80 |
| - | |
81 |
| - | |
82 |
| - | |
83 |
| - | |
84 |
| - | |
85 |
| - | |
86 |
| - | |
87 |
| - | |
88 |
| - | |
89 |
| - | |
90 |
| - | |
91 |
| - | |
92 |
| - | |
93 |
| - | |
94 |
| - | |
95 |
| - | |
96 |
| - | |
97 |
| - | |
98 |
| - | |
99 |
| - | |
100 |
| - | |
101 |
| - | |
102 |
| - | |
103 |
| - | |
104 |
| - | |
105 |
| - | |
106 |
| - | |
107 |
| - | |
108 |
| - | |
109 |
| - | |
110 |
| - | |
111 |
| - | |
112 |
| - | |
113 |
| - | |
114 |
| - | |
115 |
| - | |
116 |
| - | |
117 |
| - | |
118 |
| - | |
119 |
| - | |
120 |
| - | |
121 |
| - | |
| 78 | + | |
| 79 | + | |
| 80 | + | |
| 81 | + | |
| 82 | + | |
| 83 | + | |
| 84 | + | |
| 85 | + | |
| 86 | + | |
| 87 | + | |
| 88 | + | |
| 89 | + | |
| 90 | + | |
| 91 | + | |
| 92 | + | |
| 93 | + | |
| 94 | + | |
| 95 | + | |
| 96 | + | |
| 97 | + | |
| 98 | + | |
| 99 | + | |
| 100 | + | |
| 101 | + | |
| 102 | + | |
| 103 | + | |
| 104 | + | |
| 105 | + | |
| 106 | + | |
| 107 | + | |
| 108 | + | |
| 109 | + | |
| 110 | + | |
| 111 | + | |
| 112 | + | |
| 113 | + | |
| 114 | + | |
| 115 | + | |
| 116 | + | |
| 117 | + | |
| 118 | + | |
| 119 | + | |
| 120 | + | |
| 121 | + | |
| 122 | + | |
| 123 | + | |
| 124 | + | |
| 125 | + | |
| 126 | + | |
| 127 | + | |
| 128 | + | |
| 129 | + | |
| 130 | + | |
| 131 | + | |
122 | 132 |
| |
123 | 133 |
| |
124 | 134 |
| |
125 |
| - | |
| 135 | + | |
126 | 136 |
| |
127 | 137 |
| |
128 | 138 |
| |
129 | 139 |
| |
130 | 140 |
| |
131 | 141 |
| |
132 |
| - | |
133 |
| - | |
134 |
| - | |
135 |
| - | |
136 |
| - | |
137 |
| - | |
138 |
| - | |
139 |
| - | |
140 |
| - | |
141 |
| - | |
142 |
| - | |
143 |
| - | |
144 |
| - | |
145 |
| - | |
146 |
| - | |
147 |
| - | |
148 |
| - | |
149 |
| - | |
150 |
| - | |
151 |
| - | |
152 |
| - | |
153 |
| - | |
154 |
| - | |
155 |
| - | |
156 |
| - | |
157 |
| - | |
158 |
| - | |
159 |
| - | |
160 |
| - | |
161 |
| - | |
162 |
| - | |
163 |
| - | |
164 |
| - | |
165 |
| - | |
166 |
| - | |
167 |
| - | |
168 |
| - | |
169 |
| - | |
170 |
| - | |
171 |
| - | |
172 |
| - | |
173 |
| - | |
174 |
| - | |
175 |
| - | |
176 |
| - | |
177 |
| - | |
178 |
| - | |
179 |
| - | |
180 |
| - | |
181 |
| - | |
182 |
| - | |
183 |
| - | |
184 |
| - | |
185 |
| - | |
186 |
| - | |
187 |
| - | |
188 |
| - | |
189 |
| - | |
190 |
| - | |
191 |
| - | |
192 |
| - | |
193 |
| - | |
194 |
| - | |
195 |
| - | |
196 |
| - | |
197 |
| - | |
198 |
| - | |
199 |
| - | |
200 |
| - | |
201 |
| - | |
202 |
| - | |
203 |
| - | |
204 |
| - | |
205 |
| - | |
206 |
| - | |
207 |
| - | |
208 |
| - | |
209 |
| - | |
210 |
| - | |
211 |
| - | |
212 | 142 |
| |
213 | 143 |
| |
214 | 144 |
| |
| |||
220 | 150 |
| |
221 | 151 |
| |
222 | 152 |
| |
223 |
| - | |
224 |
| - | |
225 |
| - | |
226 |
| - | |
227 |
| - | |
228 |
| - | |
229 |
| - | |
230 |
| - | |
231 | 153 |
| |
232 | 154 |
| |
233 | 155 |
| |
| |||
248 | 170 |
| |
249 | 171 |
| |
250 | 172 |
| |
251 |
| - | |
252 |
| - | |
253 |
| - | |
254 |
| - | |
255 |
| - | |
256 |
| - | |
257 |
| - | |
258 |
| - | |
259 |
| - | |
260 |
| - | |
261 |
| - | |
262 |
| - | |
263 |
| - | |
264 |
| - | |
265 |
| - | |
266 |
| - | |
267 |
| - | |
268 |
| - | |
269 |
| - | |
270 |
| - | |
271 | 173 |
| |
272 | 174 |
| |
273 | 175 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
28 | 28 |
| |
29 | 29 |
| |
30 | 30 |
| |
31 |
| - | |
32 | 31 |
| |
33 | 32 |
| |
34 | 33 |
| |
35 |
| - | |
36 | 34 |
| |
| 35 | + | |
37 | 36 |
| |
38 | 37 |
| |
39 | 38 |
| |
40 | 39 |
| |
41 | 40 |
| |
42 | 41 |
| |
43 |
| - | |
| 42 | + | |
44 | 43 |
| |
45 | 44 |
| |
46 | 45 |
| |
| |||
65 | 64 |
| |
66 | 65 |
| |
67 | 66 |
| |
68 |
| - | |
69 |
| - | |
70 |
| - | |
71 |
| - | |
72 |
| - | |
73 |
| - | |
74 |
| - | |
75 |
| - | |
76 |
| - | |
77 |
| - | |
78 |
| - | |
79 |
| - | |
80 |
| - | |
81 |
| - | |
82 |
| - | |
83 |
| - | |
84 |
| - | |
85 |
| - | |
86 |
| - | |
87 |
| - | |
88 |
| - | |
89 |
| - | |
90 |
| - | |
91 |
| - | |
92 |
| - | |
93 |
| - | |
| 67 | + | |
| 68 | + | |
| 69 | + | |
| 70 | + | |
| 71 | + | |
| 72 | + | |
| 73 | + | |
| 74 | + | |
| 75 | + | |
| 76 | + | |
| 77 | + | |
| 78 | + | |
| 79 | + | |
| 80 | + | |
| 81 | + | |
| 82 | + | |
| 83 | + | |
| 84 | + | |
| 85 | + | |
| 86 | + | |
94 | 87 |
| |
95 |
| - | |
96 |
| - | |
97 | 88 |
| |
98 |
| - | |
99 |
| - | |
100 |
| - | |
101 |
| - | |
102 | 89 |
| |
103 |
| - | |
104 |
| - | |
105 |
| - | |
106 |
| - | |
107 |
| - | |
108 |
| - | |
109 |
| - | |
110 | 90 |
| |
111 | 91 |
| |
112 | 92 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
20 | 20 |
| |
21 | 21 |
| |
22 | 22 |
| |
| 23 | + | |
23 | 24 |
| |
24 | 25 |
| |
25 | 26 |
| |
| |||
34 | 35 |
| |
35 | 36 |
| |
36 | 37 |
| |
| 38 | + | |
37 | 39 |
| |
38 | 40 |
| |
39 | 41 |
| |
| |||
61 | 63 |
| |
62 | 64 |
| |
63 | 65 |
| |
| 66 | + | |
| 67 | + | |
| 68 | + | |
64 | 69 |
| |
65 | 70 |
| |
66 | 71 |
| |
| |||
77 | 82 |
| |
78 | 83 |
| |
79 | 84 |
| |
| 85 | + | |
| 86 | + | |
| 87 | + | |
| 88 | + | |
| 89 | + | |
| 90 | + | |
| 91 | + | |
| 92 | + | |
| 93 | + | |
| 94 | + | |
| 95 | + | |
80 | 96 |
| |
81 | 97 |
| |
82 | 98 |
| |
| |||
136 | 152 |
| |
137 | 153 |
| |
138 | 154 |
| |
| 155 | + | |
| 156 | + | |
| 157 | + | |
| 158 | + | |
| 159 | + | |
| 160 | + | |
| 161 | + | |
| 162 | + | |
| 163 | + | |
| 164 | + | |
| 165 | + | |
| 166 | + | |
| 167 | + | |
139 | 168 |
| |
140 | 169 |
| |
141 | 170 |
| |
| |||
171 | 200 |
| |
172 | 201 |
| |
173 | 202 |
| |
| 203 | + | |
| 204 | + | |
| 205 | + | |
| 206 | + | |
| 207 | + | |
| 208 | + | |
| 209 | + | |
| 210 | + | |
| 211 | + | |
174 | 212 |
| |
175 | 213 |
| |
176 | 214 |
| |
| |||
189 | 227 |
| |
190 | 228 |
| |
191 | 229 |
| |
192 |
| - | |
| 230 | + | |
| 231 | + | |
193 | 232 |
| |
194 | 233 |
| |
195 | 234 |
| |
196 | 235 |
| |
| 236 | + | |
| 237 | + | |
197 | 238 |
| |
198 | 239 |
| |
199 | 240 |
| |
200 | 241 |
| |
201 | 242 |
| |
202 | 243 |
| |
203 |
| - | |
| 244 | + | |
| 245 | + | |
204 | 246 |
| |
205 | 247 |
| |
206 | 248 |
| |
| |||
214 | 256 |
| |
215 | 257 |
| |
216 | 258 |
| |
217 |
| - | |
| 259 | + | |
| 260 | + | |
218 | 261 |
| |
219 | 262 |
| |
220 | 263 |
| |
221 | 264 |
| |
| 265 | + | |
| 266 | + | |
| 267 | + | |
222 | 268 |
| |
223 | 269 |
| |
224 | 270 |
| |
225 | 271 |
| |
226 | 272 |
| |
227 | 273 |
| |
228 |
| - | |
| 274 | + | |
| 275 | + | |
229 | 276 |
| |
230 | 277 |
| |
231 | 278 |
| |
232 | 279 |
| |
| 280 | + | |
| 281 | + | |
| 282 | + | |
| 283 | + | |
233 | 284 |
| |
234 | 285 |
| |
235 | 286 |
| |
236 | 287 |
| |
237 | 288 |
| |
238 | 289 |
| |
239 |
| - | |
| 290 | + | |
| 291 | + | |
240 | 292 |
| |
241 | 293 |
| |
242 | 294 |
| |
| |||
245 | 297 |
| |
246 | 298 |
| |
247 | 299 |
| |
248 |
| - | |
| 300 | + | |
| 301 | + | |
249 | 302 |
| |
250 | 303 |
| |
251 | 304 |
| |
252 | 305 |
| |
253 | 306 |
| |
| 307 | + | |
| 308 | + | |
| 309 | + | |
| 310 | + | |
| 311 | + | |
| 312 | + | |
| 313 | + | |
254 | 314 |
| |
255 | 315 |
| |
256 | 316 |
| |
257 | 317 |
| |
258 | 318 |
| |
| 319 | + | |
259 | 320 |
| |
260 | 321 |
| |
261 |
| - | |
| 322 | + | |
262 | 323 |
| |
263 | 324 |
| |
264 | 325 |
| |
265 | 326 |
| |
266 | 327 |
| |
267 | 328 |
| |
268 |
| - | |
| 329 | + | |
| 330 | + | |
| 331 | + | |
| 332 | + | |
| 333 | + | |
| 334 | + | |
| 335 | + | |
| 336 | + | |
| 337 | + | |
| 338 | + | |
269 | 339 |
| |
270 | 340 |
|
0 commit comments